Visual C#.NET: Read Barcode on Document & Images
How to read barcodes on document image using C#.NET
Yiigo.Imaging for .NET Barcode Reading Plugin has those following functionalities:
- Read linear & postal barcode types
- Read QR Code, Data Matrix, PDF-417 barcodes
- Get the position of the check character
- Get the bounding rectangle of the barcode
- Get error correction level of 2D barcodes
- Get the direction of recognized barcode
- Get the supplemental barcode associated with this barcode
Read Barcodes with C#.NET Sample Code Requirements
Before reading barcodes in Visual C#.NET, make sure that you have installed. Start to Read Barcodes
- Open and run your Microsoft Visual Studio;
- Choose either "Visual C# Projects" in "New Project" dialog box;
- Choose "Windows Application" in the "Templates" List and name it "YiigoImagingBarcodeReadCsharp";
- Right-click on the "References" folder, and select "Add Reference..." from the context menu in the "Solution Explorer" window;
- In the "Add Reference" dialog box, select the ".NET" tab and browse to Yiigo.Imaging.Net.dll library and Yiigo.Imaging.Net.Barcode.Read.dll ;
- Add it to your Visual C# applications;
- Call Yiigo .NET Image Namespace & Copy those following codes:
using System.IO; using System.Drawing.Printing; using Yiigo.Imaging; using Yiigo.Imaging.Processing; using Yiigo.Imaging.BarcodeRead;
// Read a barcode YiigoImage BarcodeImage = new YiigoImage("barcode.tif");
using (BarCodeRead barcode = new BarCodeRead (BarcodeImage)) { string fileName = @"C:\Users\Public\Documents\LEADTOOLS Images\Barcode.tif" ReadOpts ReadBarcode = new ReadOpts(); ReadBarcode.Direction = Directions.LeftToRight; ReadBarcode.Symbology = Symbologies.Code128; Barcode[] bars = barcode.ReadBars(options); for (int i = 0; i < bars.Length; i++) System.Console.WriteLine(bars[i].ToString()); }
// Return check digit using (BarCodeRead barcode = new BarCodeRead (BarcodeImage)) { ReadOpts ReadBarcode = new ReadOpts(); ReadBarcode.Direction = Directions.LeftToRight; ReadBarcode.Symbology = Symbologies.Code39; Barcode[] bars = barcode.ReadBars(options); for (int i = 0; i < bars.Length; i++) System.Console.WriteLine(bars[i].ToString()); }
public string ValidateMod43(string barcode) { int subtotal = 0; const string charSet = "CODE-39"; for (int i = 0; i < barcode.Length; i++) { subtotal += charSet.IndexOf(barcode.Substring(i, 1)); } return charSet.Substring(subtotal%43, 1); }
// Read barcode properties YiigoImage BarcodeImage = new YiigoImage("barcode.tif"); using (BarCodeRead barcode = new BarCodeRead (BarcodeImage) { string fileName = @"C:\Users\Public\Documents\LEADTOOLS Images\Barcode.tif" options.Direction = Directions.LeftToRight; options.Symbology = Symbologies.Code128;
Barcode[] bars = barcode.ReadBars(options); if (bars.Length == 0) { options.Direction = Directions.RightToLeft; bars = barcode.ReadBars(options); } } | 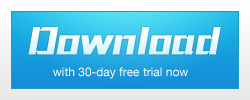
Products Overview Tech Specs Features Other Plugins Image Viewer CoreBarcode PluginPDF Read & WriteTesseract OCR PluginForm Processing PluginJBIG2 CodecJPEG2000 CodecISIS ScannerTwain ScannerDICOM ReaderCAD Scanner
|