Visual C#.NET - Image Freehand Annotation
Tutorial on How to Add Image Freehand Annotation in .NET Projects using C# Code
Yiigo.Image for .NET is one of the best SDK libraries which provides you with the complete imaging features. If you are qualified with the only requirement, i.e. that you have installed MS Visual Studio 2005 or above version / VS Express, then you can experience the wonderful image processing & annotating functionality using Visual C# and VB.NET sample codes.
In this tutorial page, users will be guided on how to draw, markup freehand annotations on images and documents such as jpeg, png, tiff, gif, pdf, etc. You will learn to draw freehand annotation & highlighter in C#, then manipulate the annotation such as visibility, size, color, font, and so on with the C# example below.
How to Add Freehand Annotation in C# Image C# Image Freehand Annotation - Requirements
- You have installed MS Visual Studio 2005 or above / MS Visual Studio Express.
- You are using .NET 2.0 or later versions.
- You are using Visual C#.NET or Visual Basic.NET as your programming language.
C# Image Freehand Annotation - Getting Started
- Download Yiigo.Image for .NET library SDK online.
- Install this C# imaging control by running the exe file from the unzipped download package.
- Type in the serial number for the purchased license to activate it. If you are simply evaluating the software, please request a free 30-day evaluation license first and then follow the wizard to activate the it.
How to Draw a C# Freehand Annotation on Image
- Start up your Microsoft Visual Studio and open your Visual C#.NET imaging project.
- Add the Yiigo annotation library named Yiigo.Image.Annotation.dll to your project reference.
- Enable the Yiigo.Image.Annotation namespace in your project, and copy the C# sample code to your imaging project. Sample image is in png image format.
- Now you can draw the freehand annotation on an image / document. Users only need to press the mouse button down, dragging (in the way you want to shape the annotation), and releasing the button.
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using Yiigo.Image.Annotation;
namespace Yiigo.Imaging.Annotation { public class FreehandLineAnnotation { public static void Test() { try { // Draw a new freehand line with annotation dll. FreeHandLineAnnotation object = new FreeHandLineAnnotation(); //set Track points object.Points = new List<LinePoint>(); object.Points.Add(new LinePoint(18.0F, 24.0F)); object.Points.Add(new LinePoint(20.3F, 1255.0F)); object.Points.Add(new LinePoint(78.0F, 144.0F));
// Adjust freehand annotation outline width object.LinePen = new LinePen(); object.LinePen.Width = 20.0F;
//set freehand annotation outline property like line style and color, object.LinePen.Brush = new AnnotationBrush(); object.LinePen.Brush.FillType = Yiigo.Imaging.Annotation.FillType.Solid; object.LinePen.Brush.Solid_Color = Color.Blue;
// Set the Closed loop property (style and color) as below if the track has Closed loop. object.Fill = new AnnotationBrush(); object.Fill.FillType = Yiigo.Imaging.Annotation.FillType.Solid;; object.Fill.Solid_Color = Color.Blue;
string folderName = "C:/"; Bitmap img = object.CreateAnnotation(); img.Save(folderName + "YiigoFreehandLineAnnotation.png"); } catch (Exception ex) { Debug.WriteLine(ex.Message); } } } }
- More image formats are supported like jpeg, gif, tiff, etc. You can also adjust annotation settings, such as flipping, rotating, resizing, text editing, etc.
- With freehand annotation you can draw annotation in sophisticated shape and also write any comment you want.
- When done, each freehand annotation can be independently resized, edited, repositioned, rotated, added to different groups, saved to system, or burned into image & documents.
C# Image Ellipse Annotation - Settings & Parameters
- Color. Color specifies the color for the freehand annotation. Default is purple.
- Width. Width specified the width for the freehand annotation. Default is 10 pixels.
C# Image Ellipse Annotation - Sample Image
Freehand Annotation Sample1 - Highlighter 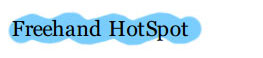
Freehand Annotation Sample2 - Custom Line & Writing 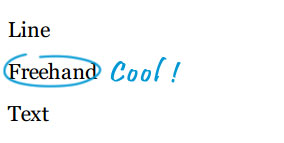
Find More C# Image Annotations Guide | 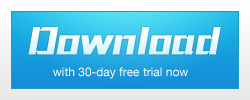
Products Overview Tech Specs Features Other Plugins Image Viewer CoreBarcode PluginPDF Read & WriteTesseract OCR PluginForm Processing PluginJBIG2 CodecJPEG2000 CodecISIS ScannerTwain ScannerDICOM ReaderCAD Scanner
|