C# Sample - Image Rubber Stamp Annotation
How to Add Rubber Stamp Annotation on Images using C# Code in .NET Projects
A rubber stamp annotation can display text or graphics which look as if they were stamped on the page/image with a rubber stamp. Yiigo image annotation library component supports several stamp text and image templates including the commonly used "Approved", "Experimental", "NotApproved", "Expired", "Confidential", "Draft" etc. Developers can also create custom rubber stamp on your own.
This page is a Visual C# tutorial for users who want to add rubber stamp feature in their .NET image project. You can follow the steps explained below to easily create a rubber stamp on your sample image (jpg/jpeg, gif, png and tiff are supported). You will also learn how to manipulate the font size, style, color, and the outline width, color, rotate / resize it, and also edit the stamp text for custom rubber stamp.
Add Rubber Stamp Annotation on Image with C# C# Image Rubber Stamp Annotation - Requirements
- .NET Framework 2.0 or later versions
- Microsoft Windows Operating System starting from Windows XP
- MS Visual Studio 2005 or later versions / MS Visual Studio Express
C# Image Rubber Stamp Annotation - Getting Started
- Download the Yiigo.Image for .NET SDK online product or trial package first.
- Unzip the SDK and run the setup file for software installation right away.
- Activate your purchased license or request an evaluation license for activation. How to activate license?
Draw C# Rubber Stamp Annotation on Images Example
- Open your Visual C#.NET imaging project in your Visual Studio or create a new one.
- Add this library from the unzipped package to your project reference: Yiigo.Image.Annotation.dll.
- Blow is the Visual C#.NET sample codes for adding a rubber stamp on your jpeg document image. And you are using the namespace Yiigo.Annotate.UI. Please copy sample coding to your project to view the result.
- Now you have printed an "Approved" rubber stamp in red on your image. You can manipulate the stamp with the parameters listed in the property section
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using Yiigo.Image.Annotation;
private void InitializeDefaultAnnotations() { // Draw a rubberstamp annotation first. RubberStampAnnotation myRubberStamp = new RubberStampAnnotation("Red Stamp"); myRubberStamp.Name = "Approved"; myRubberStamp.FontBrush.Color = Color.Red;
// Adjust the rubberstamp outline width to 15 pixels. myRubberStamp.Width = 15; myRubberStamp.OutlineColor = (#43BC6F, Color.Red);
// Specify the X and Y coordinates for lower left corner of the image rubberstamp annotation. myRubberStamp. X = 100; myRubberStamp. Y = 100;
// Set the size for the rubberstamp annotation, measured in pixel by default. myRubberStamp.Width = 150; myRubberStamp.Height = 50;
myRubberStamp.Opacity = 0; annotationController1.CurrentLayer.Items.Add(myRubberStamp); Rubber Stamp Annotation on C# Images - Some Templates
- Name: Approved. Background color: green. Background opacity: 0.5. Outline color: #43BC6F. Text value: "This document has been approved". Text align: left. Text font style: bold. Text font color: # B9C89D. Text font family: "Georgia". Font size: 20.
- Name: Rejected. Background color: red. Background opacity: 1. Outline color: #EFCDB4. Text value: "This document has been rejected". Text align: left. Text font style: italic. Text font color: # FC6173. Text font family: "Calibri". Text font size: 24.
- There are many other templates as well, such as "Expired", "Confidential", "TopSecret", "Draft", "Sold". For more information or demos, please try the rubber stamp annotation library.
C# Image Rubber Stamp Annotation - Property Settings
- By selecting a rubber stamp Name, you have selected the stamp along with its default settings. However you can manipulate it with C# coding. You can add custom rubber stamp template with your customized name.
- Outline Color specifies color for the rubber stamp. Default is red for most templates. You can adjust it with RGB value or the color palette.
- Text settings include text value, that is, the displaying text data for the actual rubber stamp.
- Text Align can control the alignment for the text data, with three modes supported - Left, Right and Center.
- Text Font settings include: 1. Style: Regular, Bold, Italic, Bold Italic. 2. Color: you can set it with RGB value or color palette. 3. Family: Calibri, Times New Roman, Arial and more. 4. Size: user-defined.
- X and Y, specify the coordinates for the lower left corner of the stamp image, used to locate stamp. Default is (0, 0).
- Width and Height refer to the rubber stamp image width and height, measured in pixel. Different default values for different rubber stamp templates. You can use these parameters to resize the annotation.
- Opacity specifies the transparency rate for the rubber stamp annotation background. If you set it to 0, then the stamp fill is completely transparent.
C# Image Rubber Stamp Annotation - Sample Image More C# Annotation Examples on Image | 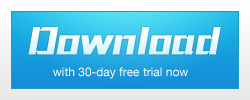
Products Overview Tech Specs Features Other Plugins Image Viewer CoreBarcode PluginPDF Read & WriteTesseract OCR PluginForm Processing PluginJBIG2 CodecJPEG2000 CodecISIS ScannerTwain ScannerDICOM ReaderCAD Scanner
|