Visual C#.NET - Image Rectangle Annotation
How to Add Rectangle Annotation on Images in .NET Projects using C# Code
Yiigo image annotation tool belongs to the Yiigo .NET imaging SDK. It supports various objects such as primitive shapes - line, circle, rectangle, polygon, and other sophisticated ones like freehand, sticky note, text, etc onto your local images.
In the sections below, C# developers & users will get to know how to draw a rectangle annotation onto your graphics using C# codings. Besides you will be able to adjust the Rectangle properties, such as outline width & color, annotation location & size, and the background color for the fill. All you need to do is simply copy the C# sample code to your project for evaluation!
How to Add Rectangle Annotation on Image using C# C# Image Rectangle Annotation - Preparation
- Check that you have installed MS Visual Studio 2005 or above / VS Express.
- Download the Yiigo.Image for .NET SDK Library by clicking the online trial button.
- Unzip the package and run the exe file for software installation.
- Type in your license serial number to activate, or request an evaluation license and activate.
Draw a C# Rectangle Annotation on Image
- Start your Visual Studio and open your C#.NET imaging project.
- Add the annotation DLL to your project reference: Yiigo.Image.Annotation.dll.
- Copy the Visual C# sample code to your project to easily create a rectangle annotation onto your png image file.
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using Yiigo.Image.Annotation;
// Draw a rectangle annotation first. RectangleAnnotation myAnnotation = new RectangleAnnotation(); myAnnotation.Text = "Rectangle Annotation";
// Set the rectangle annotation fill, i.e. to the color blue. myAnnotation.FillMode = true; myAnnotation.BackgroundColor = new AnnotationBrush(Color.LightBlue);
// Adjust the rectangle outline width to 10 pixels. myAnnotation.Width = 10; myAnnotation.OutlineColor = (128, Color.Blue);
// Specify the X and Y coordinates of the lower left corner of the rectangle annotation. myAnnotation. X = 30; myAnnotation. Y = 30;
// Now set the size for the rectangle annotation, measured in pixel by default. myAnnotation.Width = 200; myAnnotation.Height = 100;
myAnnotation.Opacity = 1; annotationController1.CurrentLayer.Items.Add(myAnnotation);
- Now you have successfully created a rectangle annotation sized 200 x 100 px on your png image, which has a non-transparent blue fill. Other formats supported include Jpeg/Jpg, Gif, Tiff images, and PDF, doc etc.
- Apart from the properties demonstrated in the Visual C# sample codes, you can also customize other rectangle annotation settings. Please check out the following property sections for more information.
C# Image Rectangle Annotation - Property Settings
- X is an int value, which sets the X coordinate for the lower left corner of the rectangle annotation to locate it.
- Y is an int value, which specifies the Y coordinate for the lower left corner of the rectangle annotation to locate it.
- Width is an int value to indicate rectangle image width, measured in pixel by default.
- Height is an int value to indicate rectangle image height, measured in pixel by default.
- Outline Color specifies the color for the rectangle outline, and the default is black [0, 0, 0]. You can customize it with RGB value or using the color palette.
- Background Color specifies the color for the rectangle fill/background, and the default is white [250, 250, 250]. Users can manipulate it with RGB value or the color palette directly. To use this property, you need to set the Fill Background property to true first.
- Fill Mode is a Boolean value indicating whether the rectangle background is filled or not.
- Line Style is the rectangle outline style, and it is Solid by default. You can select other styles such as solid line, dashed line, dotted line, dash dot line, etc.
C# Image Rectangle Annotation - Sample Image
Some More Image Annotations C# Examples | 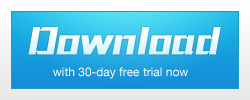
Products Overview Tech Specs Features Other Plugins Image Viewer CoreBarcode PluginPDF Read & WriteTesseract OCR PluginForm Processing PluginJBIG2 CodecJPEG2000 CodecISIS ScannerTwain ScannerDICOM ReaderCAD Scanner
|